Dynamic recipients¶
The dynamic recipients feature is a very powerful option to add custom recipients to a notification rule. It supports the syntax of Conditional templates. By using the magic wp.* or db.* functions it allows to query the database. Combined with the Placeholders of the matching post you can generate any possible kind of email list.
Note
The result of the dynamic recipients code has to be a comma separated list of email addresses.
PSN splits the resulting string by comma and checks every part if it is a valid email address. If so, the address will be added to the recipients.
Activation¶
To use this feature, you have to activate it on the PSN option tab:
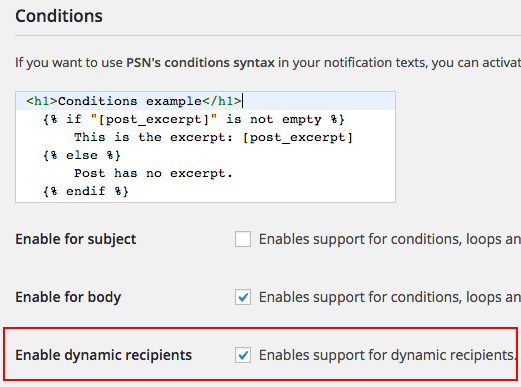
A new text editor will appear in the notification rule form:
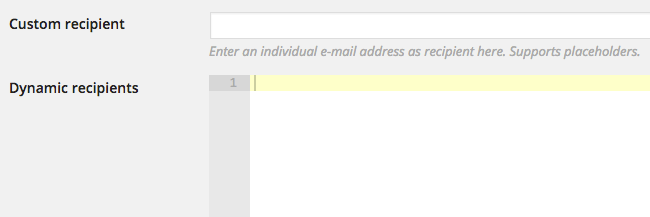
Examples¶
get_users¶

This example uses the WordPress function get_users to retrieve the email addresses of the users with the IDs 1, 2 and 3.
{% set users = wp.get_users( {"include": [1,2,3]} ) %}
{% for user in users %}
{{ user.user_email }},
{% endfor %}
Note line 3: {{ user.user_email }},
This is where the user email address gets printed in the for loop with a comma appended to it so the output will result in a list of comma separated email addresses.
Recipients depending on category¶
If the post has category “Movies” attached, the notification will be send to movie_reviewers@myblog.com. If the post has category “Games” attached, the notification will be send to games_reviewers@myblog.com. Otherwise the notification will go to default_reviewers@myblog.com.
{% if "Movies" in [post_categories_array] %}
movie_reviewers@myblog.com
{% elseif "Games" in [post_categories_array] %}
games_reviewers@myblog.com
{% else %}
default_reviewers@myblog.com
{% endif %}
SQL¶
You can use all magic db.* functions (see Magic db.* function) to run custom queries against the database.
This example uses the WordPress method get_results
(see codex.wordpress.org) to retrieve a list of users that have registered within the last month:
{% set sql = "SELECT * FROM `wp_users` WHERE `user_registered` > DATE_SUB(NOW(), INTERVAL 1 MONTH);" %}
{% set users = db.get_results(sql) %}
{% for user in users %}
{{user.user_email}},
{% endfor %}
WP Job Manager Resumes add-on¶
This is a very complex example. It allows to send a notifications about a new job listings to all resumes with the same category. The code matches the job listing category with the resume category and retrieves all resumes having that category.
The initial value is the placeholder [post_category-job_listing_category]
which contains a comma separated list of job category names. They get transformed to a list of slug names to be used in function wp.get_posts as tax_query.
Finally the custom field _candidate_email
, which keeps the resume email address, gets printed in a comma separated list.
{% set job_category_slugs = [] %}
{% set job_categories = "[post_category-job_listing_category]"|split(',') %}
{% for job_category in job_categories %}
{% set job_category_slugs = job_category_slugs|merge([wp.get_term_by("name", job_category|trim, "resume_category").slug]) %}
{% endfor %}
{% set tax_query = [{"taxonomy": "resume_category", "field": "slug", "terms": job_category_slugs|join(",")}] %}
{% set resume_args = {"post_type": "resume", "post_status": "publish", "tax_query": tax_query} %}
{% set matching_resumes = wp.get_posts(resume_args) %}
{% for resume in matching_resumes %}
{{wp.get_post_meta(resume.ID, "_candidate_email", true)}},
{% endfor %}
Matching two Custom Post Types¶
In this example we wanted to match two custom post types. When a new post with type “project” was published, we queried all posts with type “fre_profile” that have the same category like the project post. the email list gets generated by retrieving the email with wp.get_userdata with the author IDs of the matching fre_profile posts.
{% set profile_category_slugs = [] %}
{% set profile_categories = "[post_category-profile_category]"|split(',') %}
{% for profile_category in profile_categories %}
{% set profile_category_slugs = profile_category_slugs|merge([wp.get_term_by("name", profile_category|trim, "profile_category").slug]) %}
{% endfor %}
{% set tax_query = [{"taxonomy": "profile_category", "field": "slug", "terms": profile_category_slugs}] %}
{% set profile_args = {"post_type": "fre_profile", "post_status": "publish", "tax_query": tax_query} %}
{% set matching_profiles = wp.get_posts(profile_args) %}
{% for profile in matching_profiles %}
{% set user_data = wp.get_userdata(profile.post_author) %}
{{ user_data.user_email }},
{% endfor %}
The next example matches combines two queries, because using one query with relation “OR” did not work in that case.
{% set project_category_slugs = [] %}
{% set project_categories = "[post_category-project_category]"|split(',') %}
{% for project_category in project_categories %}
{% set project_category_slugs = project_category_slugs|merge([wp.get_term_by("name", project_category|trim, "project_category").slug]) %}
{% endfor %}
{% set project_skills_slugs = [] %}
{% set project_skills = "[post_category-skill]"|split(',') %}
{% for project_skill in project_skills %}
{% set project_skills_slugs = project_skills_slugs|merge([wp.get_term_by("name", project_skill|trim, "skill").slug]) %}
{% endfor %}
{% set tax_query_cat = [[{"taxonomy": "project_category", "field": "slug", "terms": project_category_slugs}]] %}
{% set profile_args_cat = {"post_type": "fre_profile", "post_status": "publish", "tax_query": tax_query_cat} %}
{% set matching_profiles_cat = wp.get_posts(profile_args_cat) %}
{% set tax_query_skill = [[{"taxonomy": "skill", "field": "slug", "terms": project_skills_slugs}]] %}
{% set profile_args_skill = {"post_type": "fre_profile", "post_status": "publish", "tax_query": tax_query_skill} %}
{% set matching_profiles_skill = wp.get_posts(profile_args_skill) %}
{% set matching_profiles_emails = [] %}
{% set matching_profiles_total = [] %}
{% set matching_profiles_total = matching_profiles_cat|merge(matching_profiles_skill) %}
{% for profile in matching_profiles_total %}
{% set user_data = wp.get_userdata(profile.post_author) %}
{% set matching_profiles_emails = matching_profiles_emails|merge([user_data.user_email]) %}
{% endfor %}
{% set matching_profiles_emails_unique = [] %}
{% for email in matching_profiles_emails %}
{% if email not in matching_profiles_emails_unique %}
{% set matching_profiles_emails_unique = matching_profiles_emails_unique|merge([email]) %}
{% endif %}
{% endfor %}
{% for email in matching_profiles_emails_unique %}
{{ email }},
{% endfor %}